这篇笔记记录Inception Net的分组卷积操作及Inception Module的作用及实现。
Google Inception Net V1首次出现在2014年,其有网络结构有22层,比同年出现的VGGNet的19层更深。Inception Net有一下特点:
- 同一层上使用多种卷积核
- 卷积核大小较小,通常只使用1x1,3x3,5x5的尺寸
Inception Module结构
其核心是分组卷积,即同一层上使用多种尺寸的卷积核,每一个卷积核得到同一层上不同尺度的特征。不同组之间的特征不交叉计算,如此便减少了计算量。
多用小尺寸的卷积核,尤其是多次用到1x1的。这是因为1x1的核性价比很高,即消耗很少的计算量就可以增加一层非线性变换。
如下图所示,实际会更灵活:
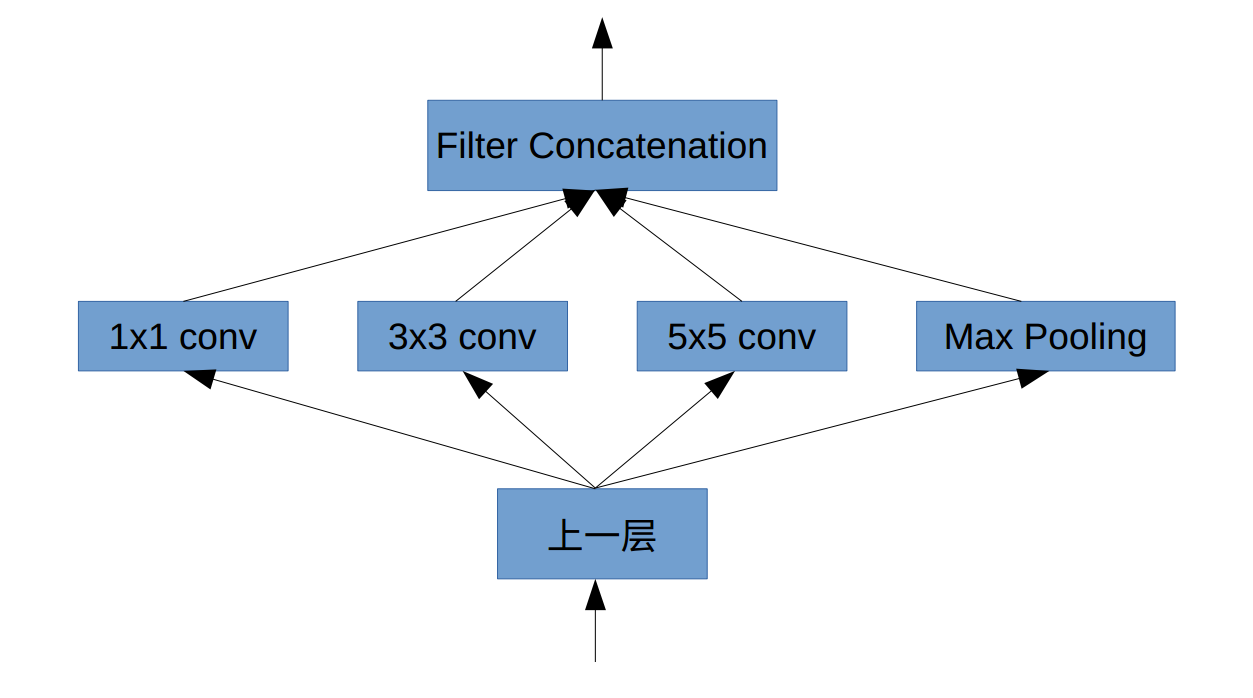
分别使用1x1, 3x3, 5x5的卷积核采样。
实现
以下是根据上图的分组卷积结构而实现的python代码:
1 | def inception_block(x, output_channel_for_each_path, name): |
以上代码片段实现的是Inception V1实际会更灵活。到了Inception V2,用两个3x3代替5x5的卷积核。到了V3,将较大的二维卷积拆成一维卷积,比如将7x7的拆成1x7和7x1两个卷积。到了V4 模型结合了ResNet的残差学习块。
本笔记记录了分组卷积的作用及实现,完整的Inception Net实现看这里。